1. Create a new “Navigation-based Application” project in XCode. In my case I have called it tabNavEg
2. Open your Application Delegate header (in my case, tabNavEgApplicationDelegate.h) and make the following changes:
a. Add UITabBarControllerDelegate as a protocol on the interface signature
b. Add tabBarController to the interface signature
c. Add a corresponding tabBarController property statement. Your Application Delegate should now look like this:
//
// tabNavEgAppDelegate.h
// tabNavEg
//
// Created by Duncan Anderson on 08/10/2010.
// Copyright __MyCompanyName__ 2010. All rights reserved.
//
#import <UIKit/UIKit.h>
#import <CoreData/CoreData.h>
@interface tabNavEgAppDelegate : NSObject
UIWindow *window;
UINavigationController *navigationController;
UITabBarController *tabBarController;
@private
NSManagedObjectContext *managedObjectContext_;
NSManagedObjectModel *managedObjectModel_;
NSPersistentStoreCoordinator *persistentStoreCoordinator_;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
@property (nonatomic, retain) IBOutlet UINavigationController *navigationController;
@property (nonatomic, retain, readonly) NSManagedObjectContext *managedObjectContext;
@property (nonatomic, retain, readonly) NSManagedObjectModel *managedObjectModel;
@property (nonatomic, retain, readonly) NSPersistentStoreCoordinator *persistentStoreCoordinator;
@property (nonatomic, retain) IBOutlet UITabBarController *tabBarController;
- (NSString *)applicationDocumentsDirectory;
@end
3. Open your Application Delegate class file (tabNavEgApplicationDelegate.m) and make the following changes:
a. Add a @synthesize tabBarController; statement
b. Replace [window addSubview:navigationController.view]; in the didFinishLaunchingWithOptions method with [window addSubview:tabBarController.view];
c. In the dealloc method add a [tabBarController release]; statement
4. Double-click MainWindow.xib to open it in Interface Builder
a. Change MainWindow.xib into list view
b. Drag a Tab Bar Controller from the library onto MainWindow.xib
c. Click on NavigationController in MainWindow.xib and rename it to FirstTabViewController
d. Drag FirstTabViewController and drop it so that it is within Tab Bar Controller
e. Click on Tab Nav Eg App Delegate in MainWindow.xib, go to the Outlets tab on the inspector, click and drag from tabBarController to connect it to the Tab Bar Controller within MainWindow.xib
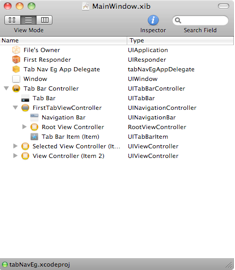
No comments :
Post a Comment